価格差・時間差の測長ツールを作成する方法【MQLプログラミングの基礎】
1.ファイルを新規作成し接頭辞を定義
この記事では、マウス操作でチャート上の距離、価格や時間を測って数値を表示する測長ツールを作る方法を解説します。ツールの機能としては、次の三つを設けます。MT4に最初から搭載されているツールの一つであるクロスヘア(十字線)に近いイメージです。
①チャート上のダブルクリックで基準点を指定し、マウスのドラッグで測定。
②ダブルクリックした位置からマウスカーソルの位置(測定点)までの差を表示。
③基準点と測定点に十字線等を表示する。
まずはファイルの新規作成で「カスタムインディケータ」を選択し、ファイル名を「MeasureTool」とします。「カスタムインディケータのイベントハンドラ」の画面では「OnChartEvent」にチェックを入れ、次の画面で「完了」をクリックすれば、ひな形の完成です。
今回はオブジェクトを使うので、ファイル上部のプロパティ「#property indicator_chart_window」の下で接頭辞「PREFIX」を定義しましょう。これを定義しておくと、オブジェクトをまとめて削除することができます。
#define PREFIX "Measure_"
またマウス操作イベントを利用するので、OnInit関数内の「//— indicator buffers mapping」の下に「CHART_EVENT_MOUSE_MOVE」を宣言しておきます。
ChartSetInteger(0, CHART_EVENT_MOUSE_MOVE, true);
2.マウスのダブルクリックを判定
今回作成するツールは、ほとんどの処理をOnChartEventの中で実行します。まずはマウスのダブルクリックを検知する回路を組んでいきましょう。マウスのX座標とY座標を定義し、位置がずれていない状態でマウスが2回押されたときに、ダブルクリックと判定とします。
CHART_EVENT_MOUSE_MOVEの場合は、「lparam」がX座標、「dparam」がY座標を示します。マウスの左ボタンのクリックや、右ボタンのクリックを判断するための数値が「sparam」として渡されます。
if (id == CHARTEVENT_MOUSE_MOVE) {
static int xPre, yPre;
int xNow = (int)lparam;
int yNow = (int)dparam;
また、一定の時間以内にマウスのボタンが押されたというのを検知するために「count」を定義し、クリックとダブルクリックの成立を記憶できるようにしておきます。
static uint count;
static bool click, dClick;
そして、sparamが「1」のときはクリックを「true」、そうでないときはクリックを「false」とします。クリックがオフのときにマウスの位置を記憶し、クリックしたときの時間を「GetTickCount」で覚えます。GetTickCountは、パソコンが起動してからの経過時間をミリ秒単位で返してくれる関数です。
GetTickCountの処理を行うのは、ダブルクリックが成立していないときとします。ダブルクリックの判定に関しては、「Now」と「Pre」が同じで座標が変わっておらず、カウントしてから200(0.2秒)以内に同じ場所で2回クリックされたかどうかが基準になります。
if (sparam == "1") {
if (!click) {
if (xNow == xPre && yNow == yPre && GetTickCount() < count + 200) dClick = true;
if (!dClick) {
xPre = xNow;
yPre = yNow;
count = GetTickCount();
}
}
click = true;
} else {
click = false;
dClick = false;
}
3.マウスの位置情報を取得
次に、ダブルクリックが成立したときに距離を測る回路を組みます。まずは「ChartXYToTimePrice」でマウスの位置のX座標、Y座標を時間情報、価格情報に変換します。失敗したときはここで処理が終了するようにします。
if (dClick) {
datetime time[2];
double price[2];
int win = 0;
if (!ChartXYToTimePrice(0, xPre, yPre, win, time[0], price[0])) return;
if (!ChartXYToTimePrice(0, xNow, yNow, win, time[1], price[1])) return;
}
これでダブルクリックした位置の情報がtime[0]とprice[0]に、今現在のマウスの位置の情報がtime[1]とprice[1]に入ります。
4.「OBJ_TREND」のサンプルコードを改修
上記では、ダブルクリックした位置の情報と、今現在のマウスの位置の情報を取得することができました。続いて、その値を利用して水平線、垂直線、トレンドラインを表示させます。
トレンドラインのサンプルコードを、MQL4リファレンスからコピーして使います。MQL4リファレンスの目次にある「Constants, Enumerations and Structures」→「Objects Constants」→「Object Types」をクリックするとオブジェクトの一覧が表示されるので、その中から「OBJ_TREND」を選択し、あらかじめ用意されている「Create a trend line by the given coordinates」のコードをコピーしてファイル下部に貼り付けます。
トレンドライン、水平線、垂直線の全てに対応できるように「// chart's ID」の「TrendCreate」を「LineCreate」に変え、「// line color」の「clr = clrRed」を「clr = clrWhite」に、「// line style」の「style = STYLE_SOLID」を「style = STYLE_DOT」に、「// highlight to move」の「selection = true」を「selection = false」に変更します。
そして、「// line name」の上にタイプのパラメーター「const int type = OBJ_TREND, // type」を追加し、「if(!ObjectCreate(chart_ID, name, OBJ_TREND, sub_window, time1, price1, time2, price2))」の「OBJ_TREND」を「type」に変えて指定できるようにしましょう。
また、「//--- set anchor points' coordinates if they are not set」から「ResetLastError();」までの4行と、「Print(__FUNCTION__,」「": failed to create a trend line! Error code = ", GetLastError());」の2行は不要なので削除してください。値が正しく反映されるように、「if(!ObjectCreate(chart_ID, name, type, sub_window, time1, price1, time2, price2))」の下に「ObjectMove(chart_ID, name, 0, time1, price1);」「ObjectMove(chart_ID, name, 1, time2, price2);」の2行を加えます。
さらにツールチップ(マウスオーバーの際に表示される補足説明)を非表示にしたいので、「ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);」の下に「ObjectSetString(chart_ID, name, OBJPROP_TOOLTIP, "\n");」も追記しましょう。
//+------------------------------------------------------------------+
//| Create a trend line by the given coordinates |
//+------------------------------------------------------------------+
bool LineCreate(const long chart_ID = 0, // chart's ID
const int type = OBJ_TREND, // type
const string name = "TrendLine", // line name
const int sub_window = 0, // subwindow index
datetime time1 = 0, // first point time
double price1 = 0, // first point price
datetime time2 = 0, // second point time
double price2 = 0, // second point price
const color clr = clrWhite, // line color
const ENUM_LINE_STYLE style = STYLE_DOT, // line style
const int width = 1, // line width
const bool back = false, // in the background
const bool selection = false, // highlight to move
const bool ray_right = false, // line's continuation to the right
const bool hidden = true, // hidden in the object list
const long z_order = 0) // priority for mouse click
{
//--- create a trend line by the given coordinates
if(!ObjectCreate(chart_ID, name, type, sub_window, time1, price1, time2, price2)) {
ObjectMove(chart_ID, name, 0, time1, price1);
ObjectMove(chart_ID, name, 1, time2, price2);
return(false);
}
//--- set line color
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
//--- set line display style
ObjectSetInteger(chart_ID, name, OBJPROP_STYLE, style);
//--- set line width
ObjectSetInteger(chart_ID, name, OBJPROP_WIDTH, width);
//--- display in the foreground (false) or background (true)
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
//--- enable (true) or disable (false) the mode of moving the line by mouse
//--- when creating a graphical object using ObjectCreate function, the object cannot be
//--- highlighted and moved by default. Inside this method, selection parameter
//--- is true by default making it possible to highlight and move the object
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
//--- enable (true) or disable (false) the mode of continuation of the line's display to the right
ObjectSetInteger(chart_ID, name, OBJPROP_RAY_RIGHT, ray_right);
//--- hide (true) or display (false) graphical object name in the object list
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
//--- set the priority for receiving the event of a mouse click in the chart
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
ObjectSetString(chart_ID, name, OBJPROP_TOOLTIP, "\n");
//--- successful execution
return(true);
}
5.「OBJ_LABEL」のサンプルコードを改修
今回は文字も表示するので、Labelオブジェクトのコードを準備します。トレンドラインと同じように、MQL4リファレンスからサンプルコードをコピーして使いましょう。
MQL4リファレンスの目次にある「Constants, Enumerations and Structures」→「Objects Constants」→「Object Types」をクリックするとオブジェクトの一覧が表示されるので、その中から「OBJ_LABEL」を選択し、あらかじめ用意されている「Create a text label」のコードをコピーしてファイル下部に貼り付けます。
「//--- reset the error value」「ResetLastError();」の2行と、「Print(__FUNCTION__,」から「return(false);」の3行は不要なので削除してください。また、「if(!ObjectCreate(chart_ID, name, OBJ_LABEL, sub_window, 0, 0))」を「ObjectCreate(chart_ID, name, OBJ_LABEL, sub_window, 0, 0)」に変更します。
//+------------------------------------------------------------------+
//| Create a text label |
//+------------------------------------------------------------------+
bool LabelCreate(const long chart_ID = 0, // chart's ID
const string name = "Label", // label name
const int sub_window = 0, // subwindow index
const int x = 0, // X coordinate
const int y = 0, // Y coordinate
const ENUM_BASE_CORNER corner = CORNER_LEFT_UPPER, // chart corner for anchoring
const string text = "Label", // text
const string font = "Arial", // font
const int font_size = 10, // font size
const color clr = clrRed, // color
const double angle = 0.0, // text slope
const ENUM_ANCHOR_POINT anchor = ANCHOR_LEFT_UPPER, // anchor type
const bool back = false, // in the background
const bool selection = false, // highlight to move
const bool hidden = true, // hidden in the object list
const long z_order = 0) // priority for mouse click
{
//--- create a text label
ObjectCreate(chart_ID, name, OBJ_LABEL, sub_window, 0, 0);
//--- set label coordinates
ObjectSetInteger(chart_ID, name, OBJPROP_XDISTANCE, x);
ObjectSetInteger(chart_ID, name, OBJPROP_YDISTANCE, y);
//--- set the chart's corner, relative to which point coordinates are defined
ObjectSetInteger(chart_ID, name, OBJPROP_CORNER, corner);
//--- set the text
ObjectSetString(chart_ID, name, OBJPROP_TEXT, text);
//--- set text font
ObjectSetString(chart_ID, name, OBJPROP_FONT, font);
//--- set font size
ObjectSetInteger(chart_ID, name, OBJPROP_FONTSIZE, font_size);
//--- set the slope angle of the text
ObjectSetDouble(chart_ID, name, OBJPROP_ANGLE, angle);
//--- set anchor type
ObjectSetInteger(chart_ID, name, OBJPROP_ANCHOR, anchor);
//--- set color
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
//--- display in the foreground (false) or background (true)
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
//--- enable (true) or disable (false) the mode of moving the label by mouse
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
//--- hide (true) or display (false) graphical object name in the object list
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
//--- set the priority for receiving the event of a mouse click in the chart
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
//--- successful execution
return(true);
}
6.水平線、垂直線、トレンドラインを表示
LineCreateを使ってラインを引いてみましょう。OnChartEvent内の「if (!ChartXYToTimePrice(0, xNow, yNow, win, time[1], price[1])) return;」の下に次のコードを記述します。「OBJ_HLINE」は水平線のことです。
LineCreate(0, OBJ_HLINE, PREFIX + "HLine0", 0, time[0], price[0], 0, 0);
これでコンパイルしてチャートにセットし、チャート上をダブルクリックすると、水平線が表示されます。
なお、ダブルクリックからのドラッグが終了したときに関連オブジェクトが消えるように、「dClick = false;」の下に次のコードも追加します。
ObjectsDeleteAll(0, PREFIX);
同様に、垂直線を引けるようにします。垂直線は「OBJ_VLINE」で表示可能です。
LineCreate(0, OBJ_VLINE, PREFIX + "VLine0", 0, time[0], price[0], 0, 0);
これでコンパイルしてチャート上をダブルクリックすると、水平線に加え垂直線も表示されることが分かります。
水平線と垂直線は測定点側にも引きたいので、そのコードを追記します。また、基準点と測定点を結ぶトレンドラインを「OBJ_TREND」で表示するようにします。
LineCreate(0, OBJ_HLINE, PREFIX + "HLine1", 0, time[1], price[1], 0, 0);
LineCreate(0, OBJ_VLINE, PREFIX + "VLine1", 0, time[1], price[1], 0, 0);
LineCreate(0, OBJ_TREND, PREFIX + "Trend", 0, time[0], price[0], time[1], price[1]);
これでコンパイルしてチャートをダブルクリックしドラッグすると、水平線、垂直線、基準点と測定点を結ぶトレンドラインが表示されます。
7.基準点から測定点までのバーの本数、時間を表示
上記では、水平線や垂直線、基準点と測定点を結ぶトレンドラインの表示ができるようになりました。次に、基準点から測定点までのバーの本数と時間を表示していきます。
バーの本数は「iBarShift」を使い、time[0]からtime[1]を引いて求めます。一方、時間は分で表示したいのでtime[1]からtime[0]を引き、それを60で割ります。
int bar = iBarShift(NULL, 0, time[0]) - iBarShift(NULL, 0, time[1]);
int min = int(time[1] - time[0]) / 60;
表示形式に関しては「バーの本数(分)」というように、バーの本数のあとに括弧付きで時間を表示します。まず「text」を定義して、LabelCreateでそれ表示します。マウスカーソルに表示が重ならないようにするために、Xの位置を「xNow + 15」として位置を少しずらしましょう。フォントは「Arial Bold」、サイズは「14」、色は「Yellow」とします。
string text = (string)bar + " (" + (string)min + "min)";
LabelCreate(0, PREFIX + "time", 0, xNow + 15, yNow, CORNER_LEFT_UPPER, text, "Arial Bold", 14, clrYellow, 0, ANCHOR_LEFT_LOWER);
これでコンパイルしてチャートをドラッグすると、基準点から測定点までのバーの本数と時間が表示されます。
8.マウスの位置の価格、基準点と測定点の価格差を表示
続いて、マウスの現在位置の価格と、基準点と測定点の価格差を「マウスの現在位置の価格(基準点と測定点の価格差)」という形式で表示するようにします。指定された数値の絶対値を返す「MathAbs」を使って価格差を求め、pipsで表示します。
double deff = MathAbs(price[0] - price[1]) / 10 / _Point;
text = DoubleToString(price[1], _Digits) + " (" + DoubleToString(deff, 1) + ")";
バーの本数、時間と同じように、LabelCreateで価格を表示します。アンカーを「ANCHOR_LEFT_UPPER」として、バーの本数と時間の下に縦に並ぶように表示しましょう。
LabelCreate(0, PREFIX + "price", 0, xNow + 15, yNow, CORNER_LEFT_UPPER, text, "Arial Bold", 14, clrYellow, 0, ANCHOR_LEFT_UPPER);
これで価格差・時間差の測長ツールは完成です。コンパイルしてチャートをドラッグすると、バーの本数と時間の下に価格情報も表示されることが分かります。
9.ソースコード
今回、作成したソースコードは下記の通りです。
//+------------------------------------------------------------------+
//| MeasureTool.mq4 |
//| Copyright 2022, MetaQuotes Software Corp. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2022, MetaQuotes Software Corp."
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
#property indicator_chart_window
#define PREFIX "Measure_"
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- indicator buffers mapping
ChartSetInteger(0, CHART_EVENT_MOUSE_MOVE, true);
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
//---
//--- return value of prev_calculated for next call
return(rates_total);
}
//+------------------------------------------------------------------+
//| ChartEvent function |
//+------------------------------------------------------------------+
void OnChartEvent(const int id,
const long &lparam,
const double &dparam,
const string &sparam)
{
if (id == CHARTEVENT_MOUSE_MOVE) {
static int xPre, yPre;
int xNow = (int)lparam;
int yNow = (int)dparam;
static uint count;
static bool click, dClick;
if (sparam == "1") {
if (!click) {
if (xNow == xPre && yNow == yPre && GetTickCount() < count + 200) dClick = true;
if (!dClick) {
xPre = xNow;
yPre = yNow;
count = GetTickCount();
}
}
click = true;
} else {
click = false;
dClick = false;
ObjectsDeleteAll(0, PREFIX);
}
if (dClick) {
datetime time[2];
double price[2];
int win = 0;
if (!ChartXYToTimePrice(0, xPre, yPre, win, time[0], price[0])) return;
if (!ChartXYToTimePrice(0, xNow, yNow, win, time[1], price[1])) return;
LineCreate(0, OBJ_HLINE, PREFIX + "HLine0", 0, time[0], price[0], 0, 0);
LineCreate(0, OBJ_VLINE, PREFIX + "VLine0", 0, time[0], price[0], 0, 0);
LineCreate(0, OBJ_HLINE, PREFIX + "HLine1", 0, time[1], price[1], 0, 0);
LineCreate(0, OBJ_VLINE, PREFIX + "VLine1", 0, time[1], price[1], 0, 0);
LineCreate(0, OBJ_TREND, PREFIX + "Trend", 0, time[0], price[0], time[1], price[1]);
int bar = iBarShift(NULL, 0, time[0]) - iBarShift(NULL, 0, time[1]);
int min = int(time[1] - time[0]) / 60;
string text = (string)bar + " (" + (string)min + "min)";
LabelCreate(0, PREFIX + "time", 0, xNow + 15, yNow, CORNER_LEFT_UPPER, text, "Arial Bold", 14, clrYellow, 0, ANCHOR_LEFT_LOWER);
double deff = MathAbs(price[0] - price[1]) / 10 / _Point;
text = DoubleToString(price[1], _Digits) + " (" + DoubleToString(deff, 1) + ")";
LabelCreate(0, PREFIX + "price", 0, xNow + 15, yNow, CORNER_LEFT_UPPER, text, "Arial Bold", 14, clrYellow, 0, ANCHOR_LEFT_UPPER);
}
}
}
//+------------------------------------------------------------------+
//| Create a trend line by the given coordinates |
//+------------------------------------------------------------------+
bool LineCreate(const long chart_ID = 0, // chart's ID
const int type = OBJ_TREND, // type
const string name = "TrendLine", // line name
const int sub_window = 0, // subwindow index
datetime time1 = 0, // first point time
double price1 = 0, // first point price
datetime time2 = 0, // second point time
double price2 = 0, // second point price
const color clr = clrWhite, // line color
const ENUM_LINE_STYLE style = STYLE_DOT, // line style
const int width = 1, // line width
const bool back = false, // in the background
const bool selection = false, // highlight to move
const bool ray_right = false, // line's continuation to the right
const bool hidden = true, // hidden in the object list
const long z_order = 0) // priority for mouse click
{
//--- create a trend line by the given coordinates
if(!ObjectCreate(chart_ID, name, type, sub_window, time1, price1, time2, price2)) {
ObjectMove(chart_ID, name, 0, time1, price1);
ObjectMove(chart_ID, name, 1, time2, price2);
return(false);
}
//--- set line color
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
//--- set line display style
ObjectSetInteger(chart_ID, name, OBJPROP_STYLE, style);
//--- set line width
ObjectSetInteger(chart_ID, name, OBJPROP_WIDTH, width);
//--- display in the foreground (false) or background (true)
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
//--- enable (true) or disable (false) the mode of moving the line by mouse
//--- when creating a graphical object using ObjectCreate function, the object cannot be
//--- highlighted and moved by default. Inside this method, selection parameter
//--- is true by default making it possible to highlight and move the object
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
//--- enable (true) or disable (false) the mode of continuation of the line's display to the right
ObjectSetInteger(chart_ID, name, OBJPROP_RAY_RIGHT, ray_right);
//--- hide (true) or display (false) graphical object name in the object list
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
//--- set the priority for receiving the event of a mouse click in the chart
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
ObjectSetString(chart_ID, name, OBJPROP_TOOLTIP, "\n");
//--- successful execution
return(true);
}
//+------------------------------------------------------------------+
//| Create a text label |
//+------------------------------------------------------------------+
bool LabelCreate(const long chart_ID = 0, // chart's ID
const string name = "Label", // label name
const int sub_window = 0, // subwindow index
const int x = 0, // X coordinate
const int y = 0, // Y coordinate
const ENUM_BASE_CORNER corner = CORNER_LEFT_UPPER, // chart corner for anchoring
const string text = "Label", // text
const string font = "Arial", // font
const int font_size = 10, // font size
const color clr = clrRed, // color
const double angle = 0.0, // text slope
const ENUM_ANCHOR_POINT anchor = ANCHOR_LEFT_UPPER, // anchor type
const bool back = false, // in the background
const bool selection = false, // highlight to move
const bool hidden = true, // hidden in the object list
const long z_order = 0) // priority for mouse click
{
//--- create a text label
ObjectCreate(chart_ID, name, OBJ_LABEL, sub_window, 0, 0);
//--- set label coordinates
ObjectSetInteger(chart_ID, name, OBJPROP_XDISTANCE, x);
ObjectSetInteger(chart_ID, name, OBJPROP_YDISTANCE, y);
//--- set the chart's corner, relative to which point coordinates are defined
ObjectSetInteger(chart_ID, name, OBJPROP_CORNER, corner);
//--- set the text
ObjectSetString(chart_ID, name, OBJPROP_TEXT, text);
//--- set text font
ObjectSetString(chart_ID, name, OBJPROP_FONT, font);
//--- set font size
ObjectSetInteger(chart_ID, name, OBJPROP_FONTSIZE, font_size);
//--- set the slope angle of the text
ObjectSetDouble(chart_ID, name, OBJPROP_ANGLE, angle);
//--- set anchor type
ObjectSetInteger(chart_ID, name, OBJPROP_ANCHOR, anchor);
//--- set color
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
//--- display in the foreground (false) or background (true)
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
//--- enable (true) or disable (false) the mode of moving the label by mouse
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
//--- hide (true) or display (false) graphical object name in the object list
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
//--- set the priority for receiving the event of a mouse click in the chart
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
//--- successful execution
return(true);
}
本記事の監修者・HT FX
2013年にFXを開始し、その後専業トレーダーへ。2014年からMT4/MT5のカスタムインジケーターの開発に取り組む。ブログでは100本を超えるインジケーターを無料公開。投資スタイルは自作の秒足インジケーターを利用したスキャルピング。
EA(自動売買)を学びたい方へオススメコンテンツ
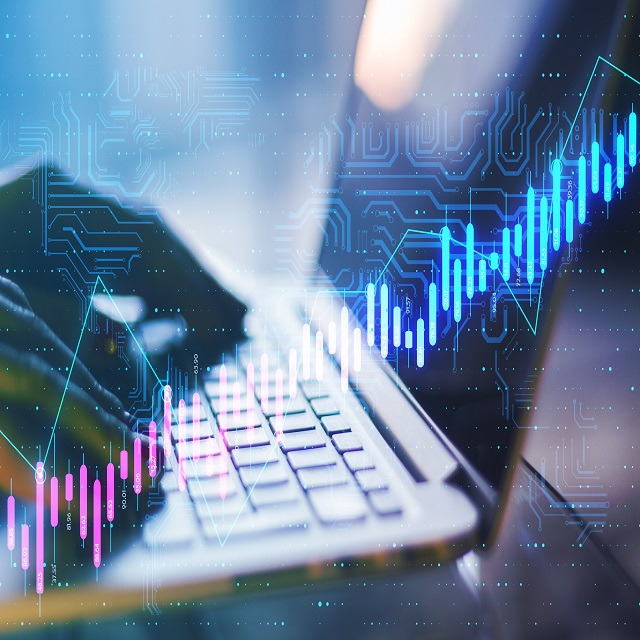
OANDAではEA(自動売買)を稼働するプラットフォームMT4/MT5の基本的な使い方について、画像や動画付きで詳しく解説しています。MT4/MT5のインストールからEAの設定方法までを詳しく解説しているので、初心者の方でもスムーズにEA運用を始めることが可能です。またOANDAの口座をお持ちであれば、独自開発したオリジナルインジケーターを無料で利用することもできます。EA運用をお考えであれば、ぜひ口座開設をご検討ください。
本ホームページに掲載されている事項は、投資判断の参考となる情報の提供を目的としたものであり、投資の勧誘を目的としたものではありません。投資方針、投資タイミング等は、ご自身の責任において判断してください。本サービスの情報に基づいて行った取引のいかなる損失についても、当社は一切の責を負いかねますのでご了承ください。また、当社は、当該情報の正確性および完全性を保証または約束するものでなく、今後、予告なしに内容を変更または廃止する場合があります。なお、当該情報の欠落・誤謬等につきましてもその責を負いかねますのでご了承ください。